参考书籍:《C#程序设计实验指导与习题测试》
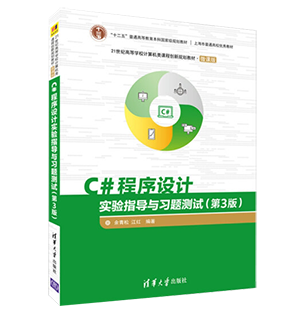
- 掌握类的声明和对象的创建
- 掌握对象的封装性
- 掌握类的访问修饰符
- 掌握类的继承的实现
- 掌握派生类、抽象类、抽象方法的使用
- 掌握类的多态性
- 掌握运算符的重载
- 了解接口的实现
- 了解事件的实现
实验1:创建类MyMath,计算圆的周长、面积和球的体积
创建类MyMath,包含常量PI,静态方法Perimeter(周长)、Area(面积)、Volume(体积)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| using System;
namespace ConsoleApp5 { class MyMath { public const double PI = 3.1415926; public static double Perimeter (double r) { double p = 2 * PI * r; return p; } public static double Area (double r) { double a = PI * r * r; return a; } public static double Volume (double r) { double v = (4 * PI * r * r * r) / 3; return v; } } class Program { static void Main(string[] args) { Console.Write("请输入半径:"); double r = double.Parse(Console.ReadLine()); Console.WriteLine("圆的周长为:{0}",MyMath.Perimeter(r)); Console.WriteLine("圆的面积为:{0}",MyMath.Area(r)); Console.WriteLine("对应球的体积为:{0}",MyMath.Volume(r)); Console.ReadKey(); } } }
|
1 2 3 4
| 请输入半径:5 圆的周长为:31.415926 圆的面积为:78.539815 对应球的体积为:523.5987666666666
|
实验2:创建表示摄氏温度的类TemperatureCelsius
创建类TemperatureCelsius,包含实力字段degree(表示摄氏温度)和实例方法ToFahrenheit(将摄氏温度转换成华氏温度)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| using System;
namespace ConsoleApp5 { public class TemperatrueCelsius { protected double degree; public TemperatrueCelsius(double d) { this.degree = d; } public double ToFahrenheit() { return (degree * 9 / 5) + 32; } }
class Program { static void Main(string[] args) { Console.Write("请输入摄氏温度:"); double d = Double.Parse(Console.ReadLine()); TemperatrueCelsius celsius = new TemperatrueCelsius(d); Console.WriteLine("摄氏温度={0},华氏温度={1}", d, celsius.ToFahrenheit()); Console.ReadKey(); } } }
|
1 2
| 请输入摄氏温度:15 摄氏温度=15,华氏温度=59
|
实验3:类的继承的实现
创建积累Person和派生类Teacher。基类Person包含实例字段name和age;虚函数GetInfo()显示个人信息。派生类Teacher除了包含基类的name和age字段外,还包含自己的TeacherID字段,并使用关键字override来重写方法GetInfo()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| using System;
namespace ConsoleApp5 { public class Person { public string name; public uint age; public Person(String name,uint age) { this.name = name; this.age = age; } public virtual void GetInfo() { Console.WriteLine("Name:{0}", name); Console.WriteLine("Age:{0}",age); } } public class Teacher:Person { public string teacherID; public Teacher(String name,uint age,string id) :base(name,age) { this.teacherID = id; } public override void GetInfo() { base.GetInfo(); Console.WriteLine("teacherID:{0}", teacherID); } } class Program { static void Main(string[] args) { Teacher objteacher = new Teacher("Mr.Yu", 40, "1990108001"); objteacher.GetInfo(); Console.ReadKey(); } } }
|
1 2 3
| Name:Mr.Yu Age:40 teacherID:1990108001
|
实验4:抽象类、抽象方法、多态性的实现
创建抽象基类Shape和派生类Rectangle、circle。利用多态性实现Area(计算面积)和Show(显示图形名称和面积)抽象方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| using System;
namespace ConsoleApp5 { public abstract class Shape { protected string name;
public Shape(string name) { this.name = name; } public abstract void Show(); public abstract double Area(); } public class Rectangle:Shape { protected double weight; protected double height; public Rectangle (string name,double w,double h) :base(name) { this.weight = w; this.height = h; } public override void Show() { Console.WriteLine("Rectangle:{0}\nArea:{1}",name,weight*height); } public override double Area() { return weight * height; } } public class Circle:Shape { protected double radius; public Circle (String name,double r) :base(name) { this.radius = r; } public override void Show() { Console.WriteLine("Circle:{0}\nArea:{1}", name,Math.PI* radius* radius); } public override double Area() { return Math.PI * radius * radius; } } class Program { static void Main(string[] args) { Shape[] s = { new Rectangle("矩形", 1.0, 2.0), new Circle("圆", 3.5) }; foreach(Shape e in s) { e.Show(); } Console.ReadKey(); } } }
|
1 2 3 4
| Rectangle:矩形 Area:2 Circle:圆 Area:38.48451000647496
|
实验5:运算符重载
使用运算符重载,创建定义复数相加、相减和相乘的复数类Complex。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| using System;
namespace ConsoleApp5 { public struct Complex { public int real; public int imaginary; public Complex(int real,int imaginary) { this.real = real; this.imaginary = imaginary; } public static Complex operator +(Complex c1,Complex c2) { return new Complex(c1.real + c2.real, c1.imaginary+c2.imaginary); } public static Complex operator -(Complex c1, Complex c2) { return new Complex(c1.real - c2.real, c1.imaginary - c2.imaginary); } public static Complex operator *(Complex c1, Complex c2) { return new Complex(c1.real * c2.real-c1.imaginary * c2.imaginary, c1.real * c2.imaginary + c1.imaginary * c2.real); } public override string ToString() { return (String.Format("{0}+{1}i", real, imaginary)); } } class TestComplex { static void Main() { Complex num1 = new Complex(4, 5); Complex num2 = new Complex(3, 2); Complex sum = num1 + num2; Complex sub = num1 - num2; Complex mul = num1 * num2; Console.WriteLine("第一个复数:{0}", num1); Console.WriteLine("第二个复数:{0}", num2); Console.WriteLine("两个复数之和:{0}", sum); Console.WriteLine("两个复数之差:{0}", sub); Console.WriteLine("两个复数之积:{0}", mul); Console.ReadKey(); } } }
|
1 2 3 4 5
| 第一个复数:4+5i 第二个复数:3+2i 两个复数之和:7+7i 两个复数之差:1+3i 两个复数之积:2+23i
|
实验6:接口的实现
声明一个接口ICDPlayer,包含4个借口方法:Play()、Stop()、NextTrack()和PreviousTrack(),以及一个只读属性CurrentTrack,创建类CDPlayer实现该接口,模拟CD的播放,停止、下一个音轨、上一音轨的操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| using System;
namespace ConsoleApp5 { public interface ICDPlayer { void Play(); void Stop(); void PreviousTrack(); void NextTrack(); int CurrentTrack { get; } } public class CDPlayer: ICDPlayer { private int currentTrack = 0; public void Play() { Console.WriteLine("启动CD..."); } public void Stop() { Console.WriteLine("停止CD..."); } public void PreviousTrack() { Console.WriteLine("前一个音轨..."); if(currentTrack>=1) { currentTrack--; } } public void NextTrack() { Console.WriteLine("后一个音轨..."); currentTrack++; } public int CurrentTrack { get { return currentTrack; } } } class TestCDPlayer { static void Main() { CDPlayer myCD = new CDPlayer(); myCD.Play(); Console.WriteLine("myCD.CurrentTrack={0}", myCD.CurrentTrack); myCD.NextTrack(); myCD.NextTrack(); Console.WriteLine("myCD.CurrentTrack={0}", myCD.CurrentTrack); ICDPlayer myICD = (ICDPlayer)myCD; myICD.PreviousTrack(); Console.WriteLine("myICD.CurrentTrack={0}", myCD.CurrentTrack); myICD.Stop(); Console.ReadKey(); } } }
|
1 2 3 4 5 6 7 8
| 启动CD... myCD.CurrentTrack=0 后一个音轨... 后一个音轨... myCD.CurrentTrack=2 前一个音轨... myICD.CurrentTrack=1 停止CD...
|
实验7:事件的实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| using System; using System.Collections; namespace ConsoleApp5 { public class NameListEventArgs:EventArgs { public string Name { get; set; } public int Count { get; set; } public NameListEventArgs(string name ,int count) { Name = name; Count = count; } } public delegate void NameListEventHandler(object source, NameListEventArgs args); public class NameList { ArrayList list; public event NameListEventHandler nameListEvent; public NameList() { list = new ArrayList(); } public void Add(string Name) { list.Add(Name); if(nameListEvent != null) { nameListEvent(this, new NameListEventArgs(Name, list.Count)); } } } public class EventDemo { public static void Method1(object source,NameListEventArgs args) { Console.WriteLine("列表中增加了项目:{0}", args.Name); } public static void Method2(object source, NameListEventArgs args) { Console.WriteLine("列表中的项目数:{0}", args.Count); } public static void Main() { NameList n1 = new NameList(); n1.nameListEvent += new NameListEventHandler(EventDemo.Method1); n1.nameListEvent += new NameListEventHandler(EventDemo.Method2); n1.Add("张三"); n1.Add("李四"); n1.Add("王五"); Console.ReadKey(); } }
}
|
1 2 3 4 5 6
| 列表中增加了项目:张三 列表中的项目数:1 列表中增加了项目:李四 列表中的项目数:2 列表中增加了项目:王五 列表中的项目数:3
|